Note
Go to the end to download the full example code
Wrapping Other Objects#
You can wrap several other object types using pyvista including:
numpy arrays
trimesh.Trimesh meshes
VTK objects
This allows for the “best of both worlds” programming special to Python due to its modularity. If there’s some limitation of pyvista (or trimesh), then you can adapt your scripts to use the best features of more than one module.
Wrap a point cloud composed of random points from numpy
import numpy as np
import pyvista as pv
points = np.random.random((30, 3))
cloud = pv.wrap(points)
pv.plot(
cloud,
scalars=points[:, 2],
render_points_as_spheres=True,
point_size=50,
opacity=points[:, 0],
cpos='xz',
)
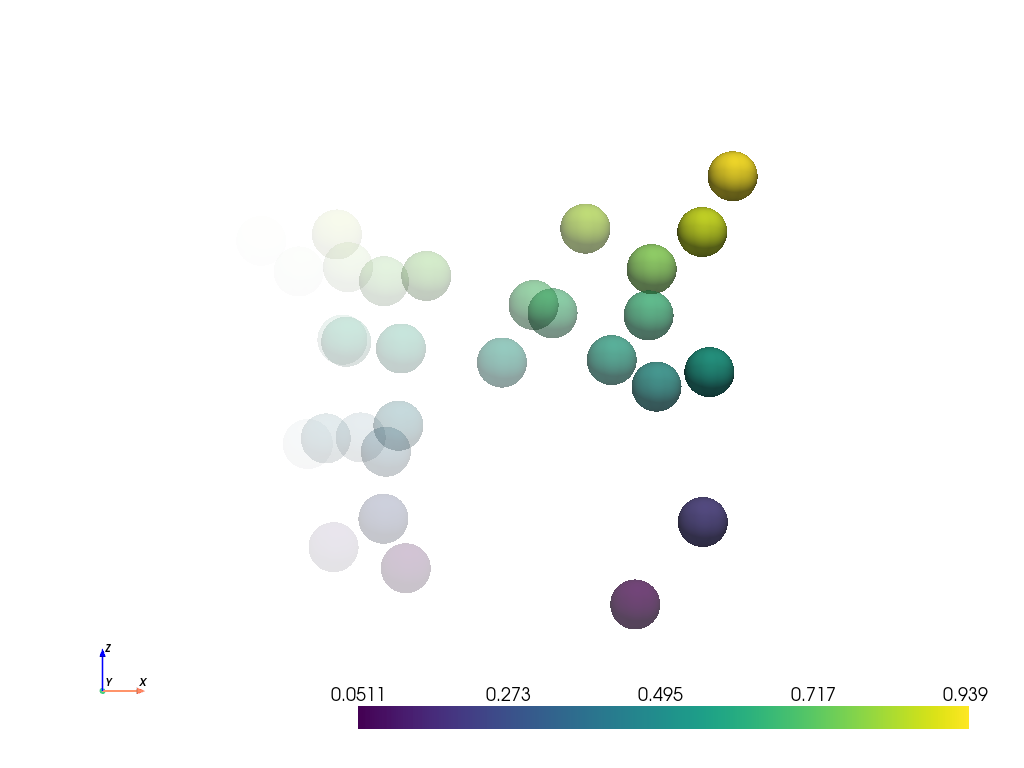
Wrap an instance of Trimesh
PolyData (0x7fd382bfe5c0)
N Cells: 1
N Points: 3
N Strips: 0
X Bounds: 0.000e+00, 0.000e+00
Y Bounds: 0.000e+00, 1.000e+00
Z Bounds: 0.000e+00, 1.000e+00
N Arrays: 0
Wrap an instance of vtk.vtkPolyData
PolyData (0x7fd38287fa60)
N Cells: 1
N Points: 1
N Strips: 0
X Bounds: 1.000e+00, 1.000e+00
Y Bounds: 2.000e+00, 2.000e+00
Z Bounds: 3.000e+00, 3.000e+00
N Arrays: 0
Total running time of the script: (0 minutes 0.861 seconds)