Note
Go to the end to download the full example code.
Decimation#
Decimate a mesh
from __future__ import annotations
import numpy as np
import pyvista as pv
from pyvista import examples
mesh = examples.download_face()
# Define a camera position that shows this mesh properly
cpos = [(0.4, -0.07, -0.31), (0.05, -0.13, -0.06), (-0.1, 1, 0.08)]
dargs = dict(show_edges=True, color=True)
# Preview the mesh
mesh.plot(cpos=cpos, **dargs)
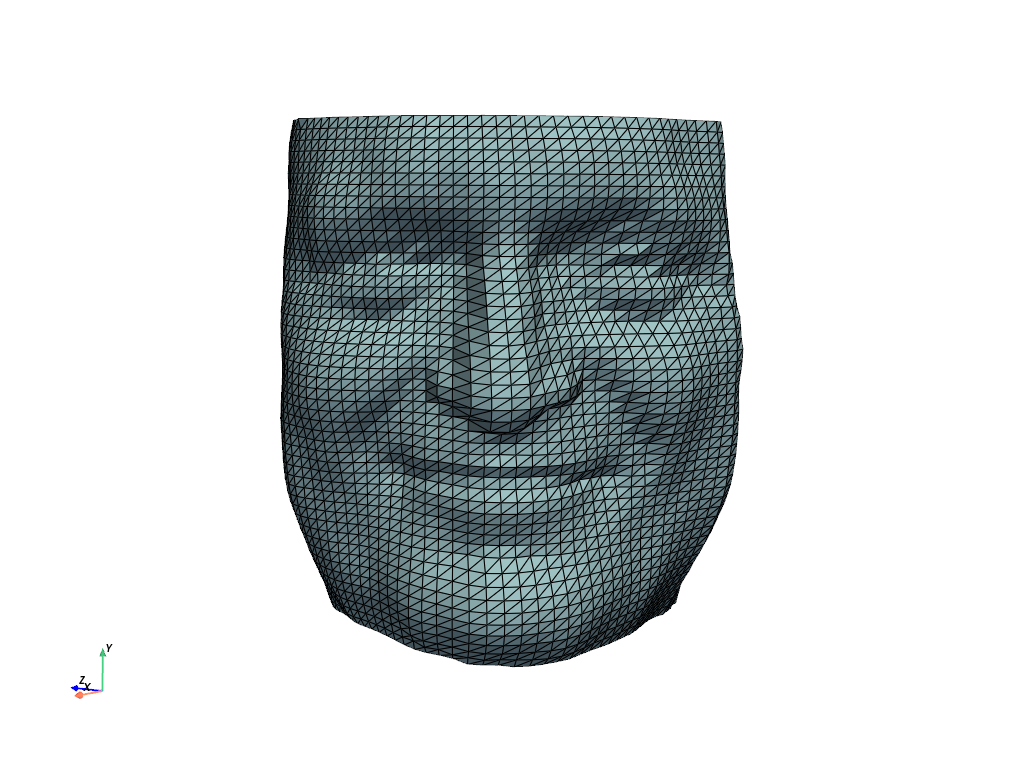
Now let’s define a target reduction and compare the
pyvista.PolyDataFilters.decimate()
and
pyvista.PolyDataFilters.decimate_pro()
filters.
target_reduction = 0.7
print(f'Reducing {target_reduction * 100.0} percent out of the original mesh')
Reducing 70.0 percent out of the original mesh
decimated = mesh.decimate(target_reduction)
decimated.plot(cpos=cpos, **dargs)
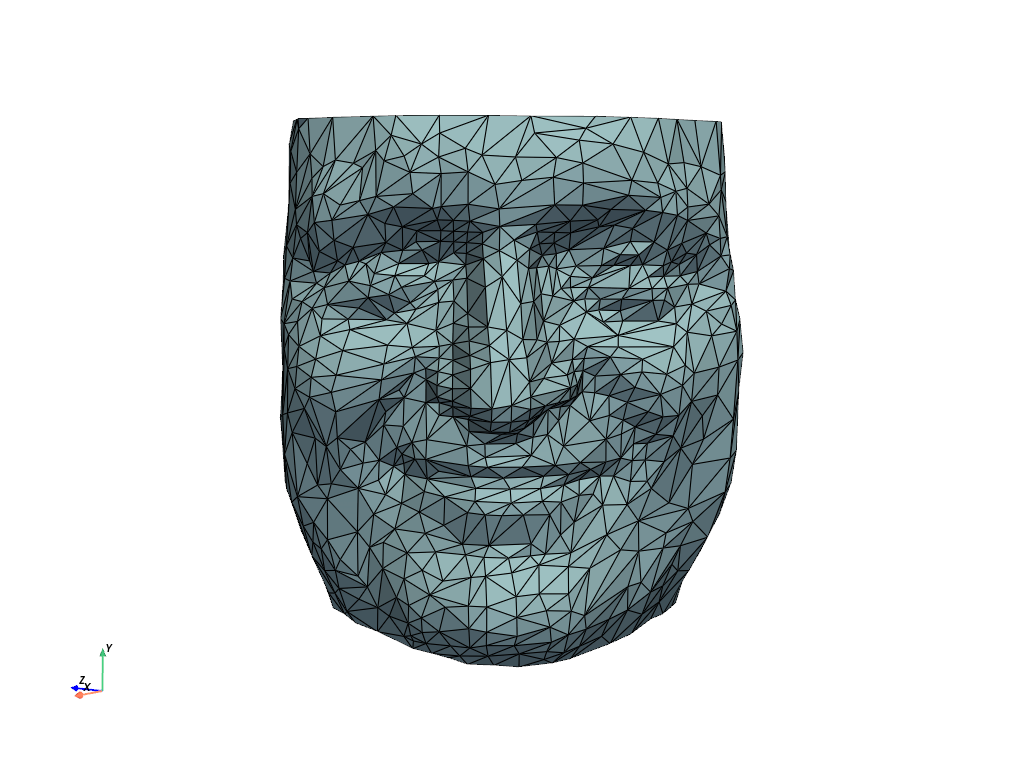
pro_decimated = mesh.decimate_pro(target_reduction, preserve_topology=True)
pro_decimated.plot(cpos=cpos, **dargs)
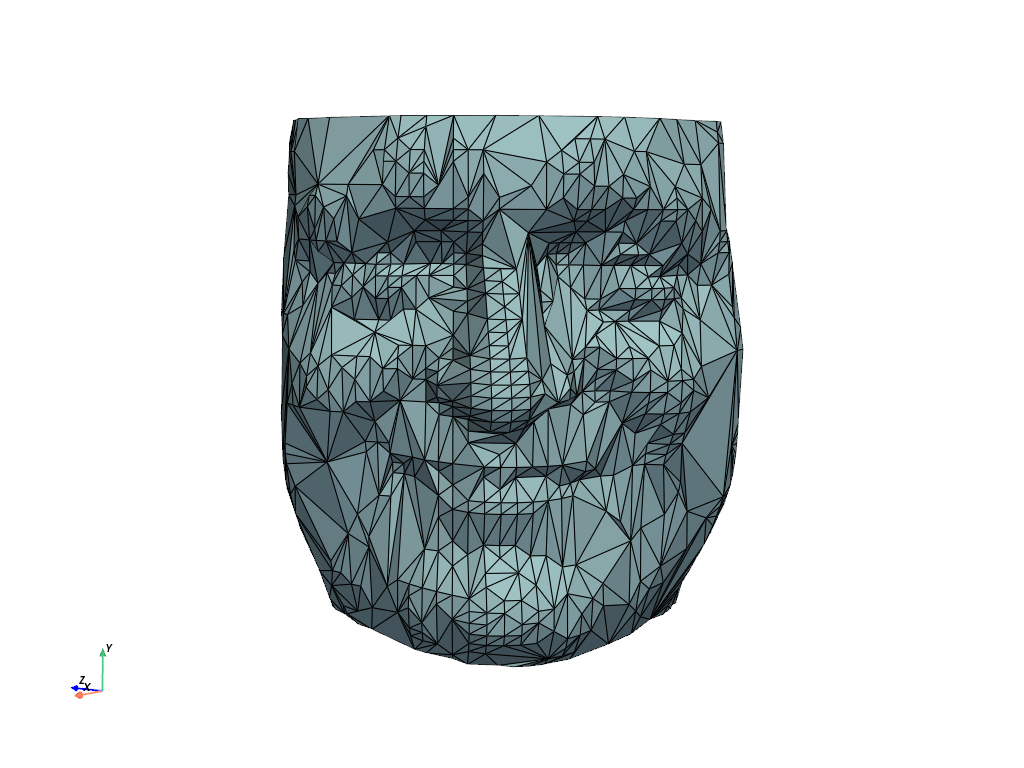
Side by side comparison:
pl = pv.Plotter(shape=(1, 3))
pl.add_mesh(mesh, **dargs)
pl.add_text('Input mesh', font_size=24)
pl.camera_position = cpos
pl.reset_camera()
pl.subplot(0, 1)
pl.add_mesh(decimated, **dargs)
pl.add_text('Decimated mesh', font_size=24)
pl.camera_position = cpos
pl.reset_camera()
pl.subplot(0, 2)
pl.add_mesh(pro_decimated, **dargs)
pl.add_text('Pro Decimated mesh', font_size=24)
pl.camera_position = cpos
pl.reset_camera()
pl.link_views()
pl.show()
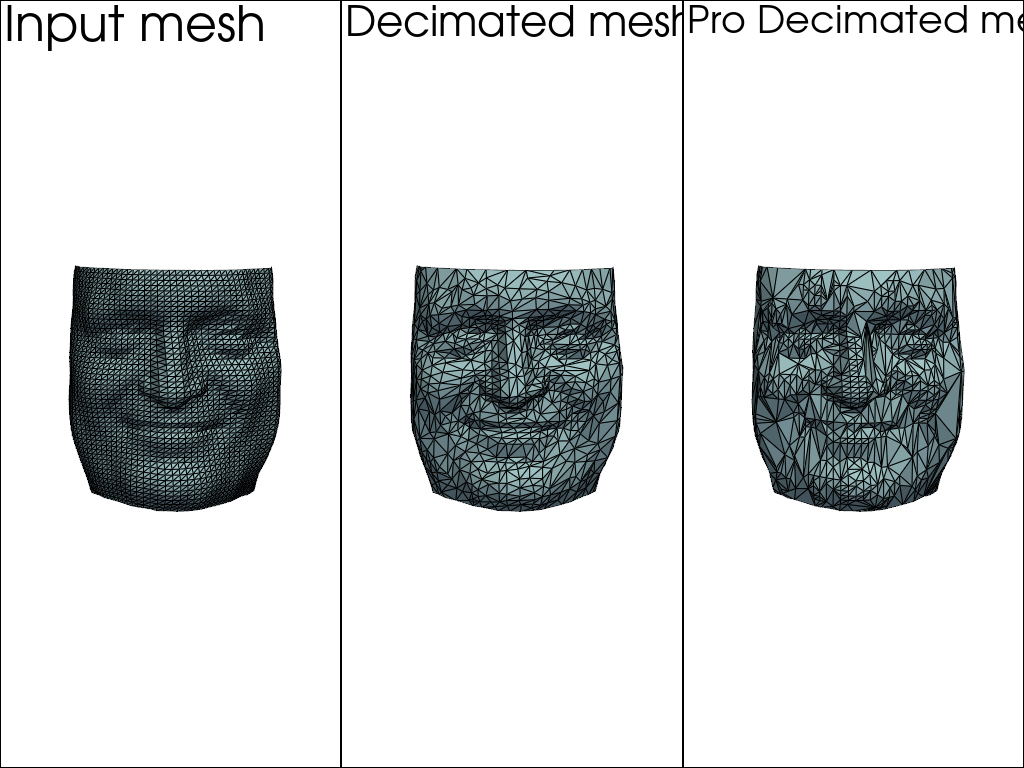
Decimate Polyline Mesh#
Generate a fairly slow spiral polyline mesh.
Construct a reusable plotting function for future use.
def compare_decimation(spiral, decimated):
pl = pv.Plotter()
pl.add_mesh(spiral, line_width=5, color='r', label='Original')
pl.add_mesh(decimated, line_width=3, color='k', label='Decimated')
pl.view_xy()
pl.add_legend(face='line', size=(0.25, 0.25))
Decimate using pyvista.PolyDataFilters.decimate_polyline()
filter by
target of 50%.
Original # of points: 100
Decimated # of points: 50
The decimation looks OK at this level of reduction.
compare_decimation(spiral, decimated)
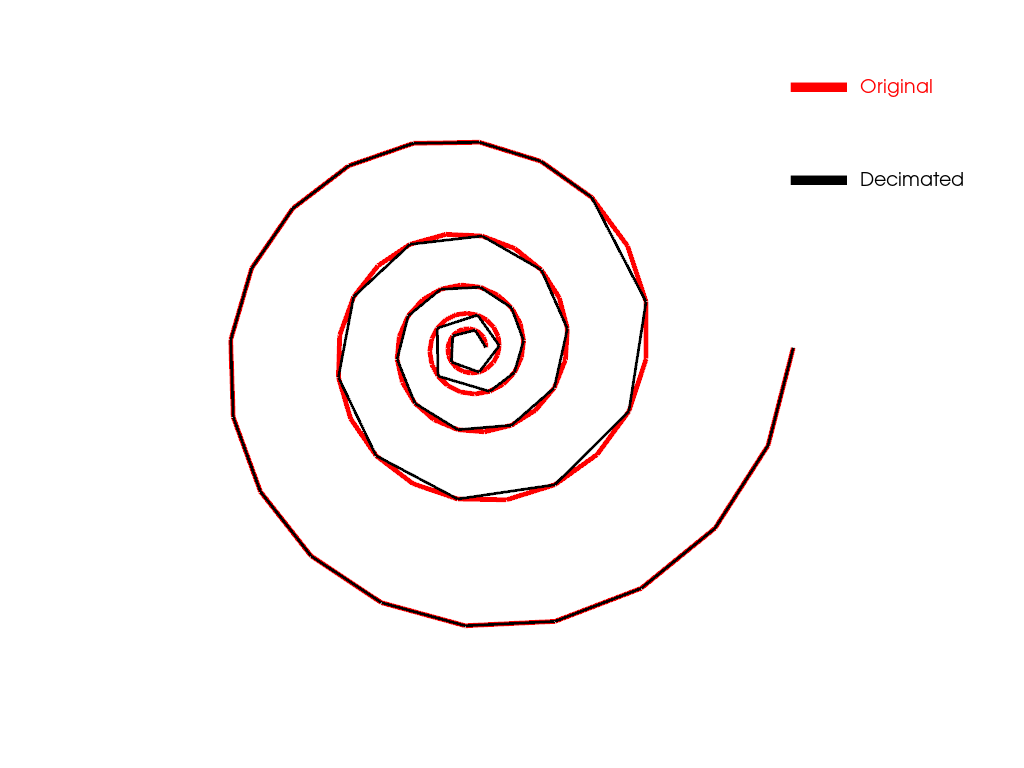
Using a larger level of reduction, 80%, leads to a much coarser level of representation.
Original # of points: 100
Decimated # of points: 20
The structure of the inner part of the spiral is completely lost.
compare_decimation(spiral, decimated)
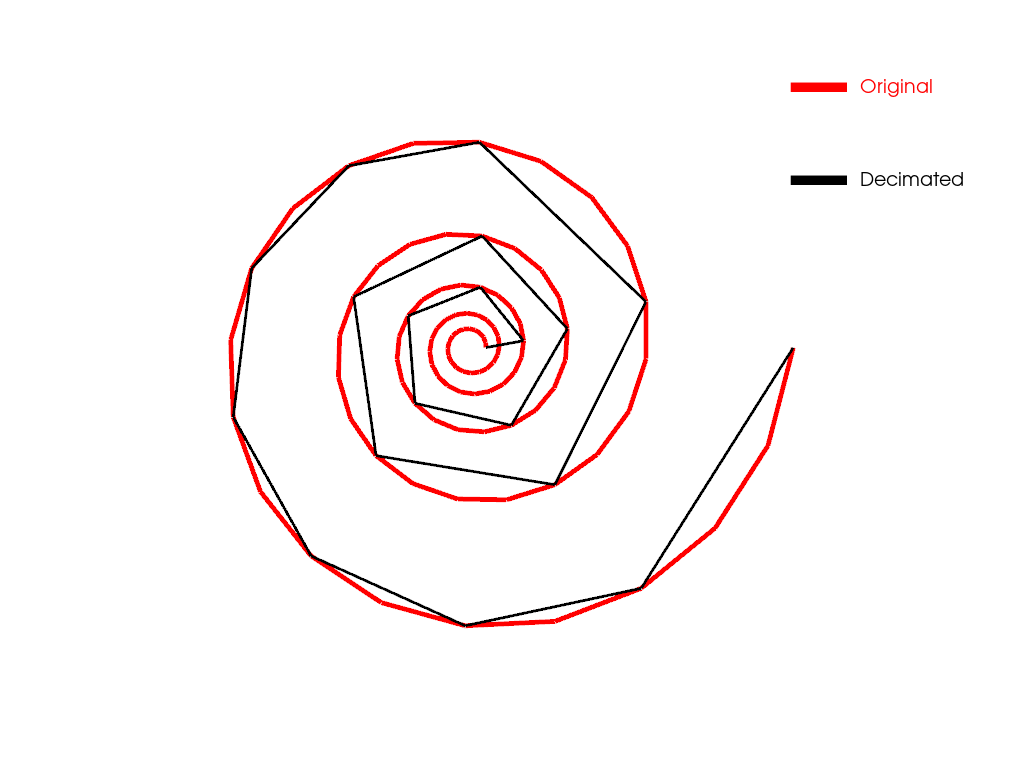
To avoid errors of quickly changing features, use the maximum_error
parameter. It is in units of fraction of the largest length of the
bounding box. Note that it limits the level of reduction achieved.
Original # of points: 100
Decimated # of points: 45
The structure of the inner part of the spiral is captured adequately.
compare_decimation(spiral, decimated)
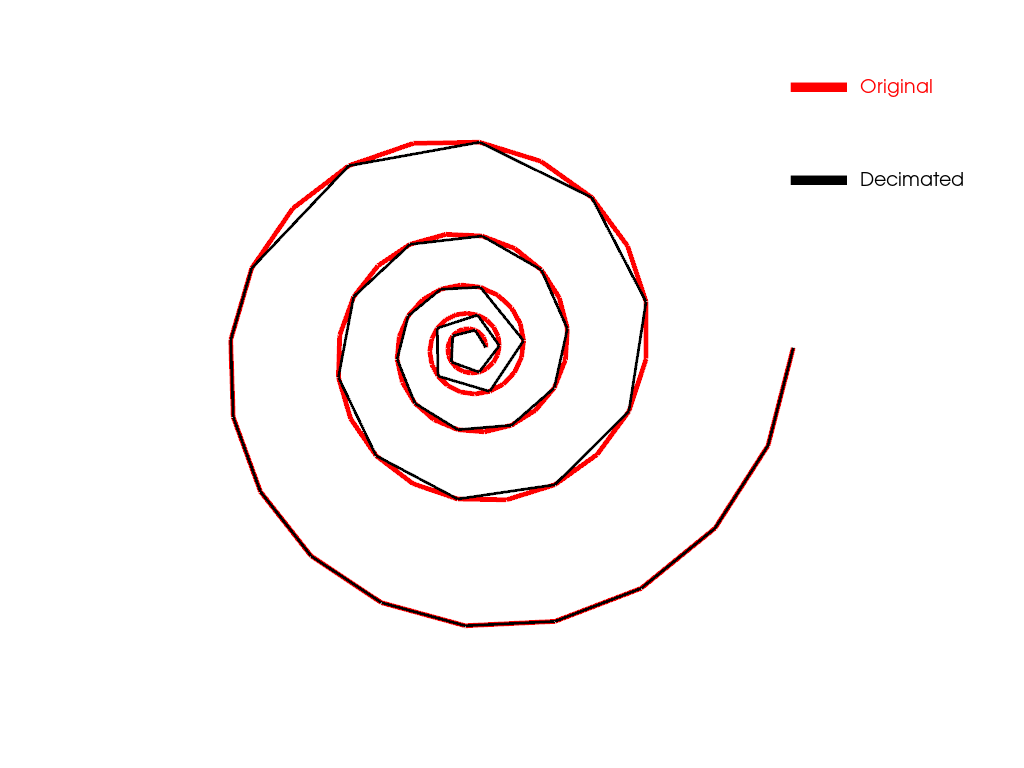
Total running time of the script: (0 minutes 1.339 seconds)