Cells#
The cell pyvista.Cell
class is the PyVista representation of the
vtkGenericCell and can be used to inspect a pyvista.DataSet
’s cells, faces, and edges.
Note
While methods and classes are quite effective at inspecting and plotting
parts of a dataset, they are inefficient and should be used only for
interactive exploration and debugging. When working with larger datasets or
working with multiple cells it is generally more efficient to use bulk methods
like pyvista.DataSetFilters.extract_cells()
.
Here’s a quick example to demonstrate the usage of pyvista.DataSet.get_cell()
by extracting a hexahedral cell from an example pyvista.UnstructuredGrid
.
from pyvista import examples
mesh = examples.load_hexbeam()
cell = mesh.get_cell(0)
cell
Cell (0x7ff248751f00)
Type: <CellType.HEXAHEDRON: 12>
Linear: True
Dimension: 3
N Points: 8
N Faces: 6
N Edges: 12
X Bounds: 0.000e+00, 5.000e-01
Y Bounds: 0.000e+00, 5.000e-01
Z Bounds: 0.000e+00, 5.000e-01
You can then extract a single face of that cell.
face = cell.get_face(0)
face
Cell (0x7ff248752260)
Type: <CellType.QUAD: 9>
Linear: True
Dimension: 2
N Points: 4
N Faces: 0
N Edges: 4
X Bounds: 0.000e+00, 0.000e+00
Y Bounds: 0.000e+00, 5.000e-01
Z Bounds: 0.000e+00, 5.000e-01
Afterwards, you can extract an edge or edges from the face.
edge = face.get_edge(0)
edge
Cell (0x7ff248752140)
Type: <CellType.LINE: 3>
Linear: True
Dimension: 1
N Points: 2
N Faces: 0
N Edges: 0
X Bounds: 0.000e+00, 0.000e+00
Y Bounds: 0.000e+00, 0.000e+00
Z Bounds: 0.000e+00, 5.000e-01
Each
pyvista.Cell
can be individually plotted for convenience.cell.plot(show_edges=True, line_width=3)
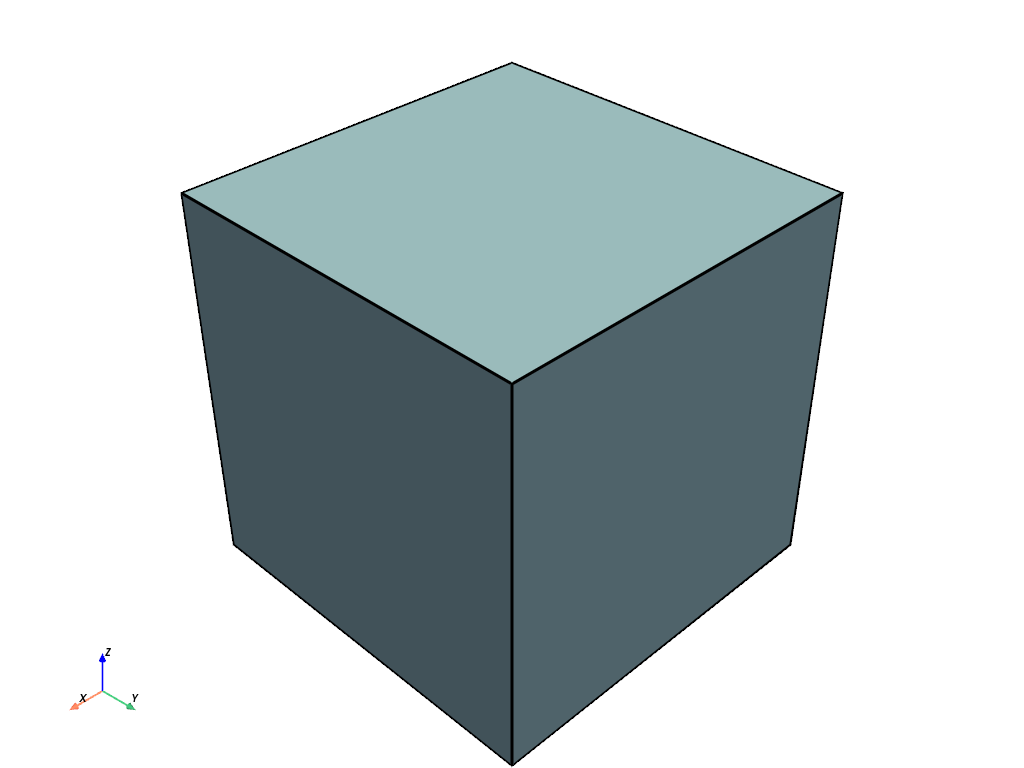
Class Definition#
|
Wrapping of vtkCell. |