Note
Go to the end to download the full example code.
Label based on Distance on Line#
Create a spline and generate labels along the spline based on distance along a spline.
This is an extension of the Creating a Spline.
from __future__ import annotations
import numpy as np
import pyvista as pv
Create a spline#
Create a spline using pyvista.Spline()
.
# Make points along a spline
theta = np.linspace(-1 * np.pi, 1 * np.pi, 100)
z = np.linspace(2, -2, 100)
r = z**2 + 1
x = r * np.sin(theta)
y = r * np.cos(theta)
points = np.column_stack((x, y, z))
# Create a spline. This automatically computes arc_length, which is the
# distance along the line.
spline = pv.Spline(points, 1000)
spline.point_data
pyvista DataSetAttributes
Association : POINT
Active Scalars : None
Active Vectors : None
Active Texture : None
Active Normals : None
Contains arrays :
arc_length float32 (1000,)
Determine the coordinates matching distance along a spline#
Here we write a simple function that gets the closest point matching a distance along a spline and then generate labels for those points.
def get_point_along_spline(distance):
"""Return the closest point on the spline given a length along the spline."""
idx = np.argmin(np.abs(spline.point_data['arc_length'] - distance))
return spline.points[idx]
# distances along the spline we're interested in
dists = [0, 4, 8, 11]
# make labels
labels = []
label_points = []
for dist in dists:
point = get_point_along_spline(dist)
labels.append(f'Dist {dist}: ({point[0]:.2f}, {point[1]:.2f}, {point[2]:.2f})')
label_points.append(point)
labels
['Dist 0: (-0.00, -5.00, 2.00)', 'Dist 4: (-2.36, -2.00, 1.45)', 'Dist 8: (-0.59, 0.96, 0.35)', 'Dist 11: (1.85, 0.20, -0.93)']
Plot with Labels#
Plot the spline with labeled points
pl = pv.Plotter()
pl.add_mesh(spline, scalars='arc_length', render_lines_as_tubes=True, line_width=10)
pl.add_point_labels(
label_points,
labels,
always_visible=True,
point_size=20,
render_points_as_spheres=True,
)
pl.show_bounds()
pl.show_axes()
pl.camera_position = 'xz'
pl.show()
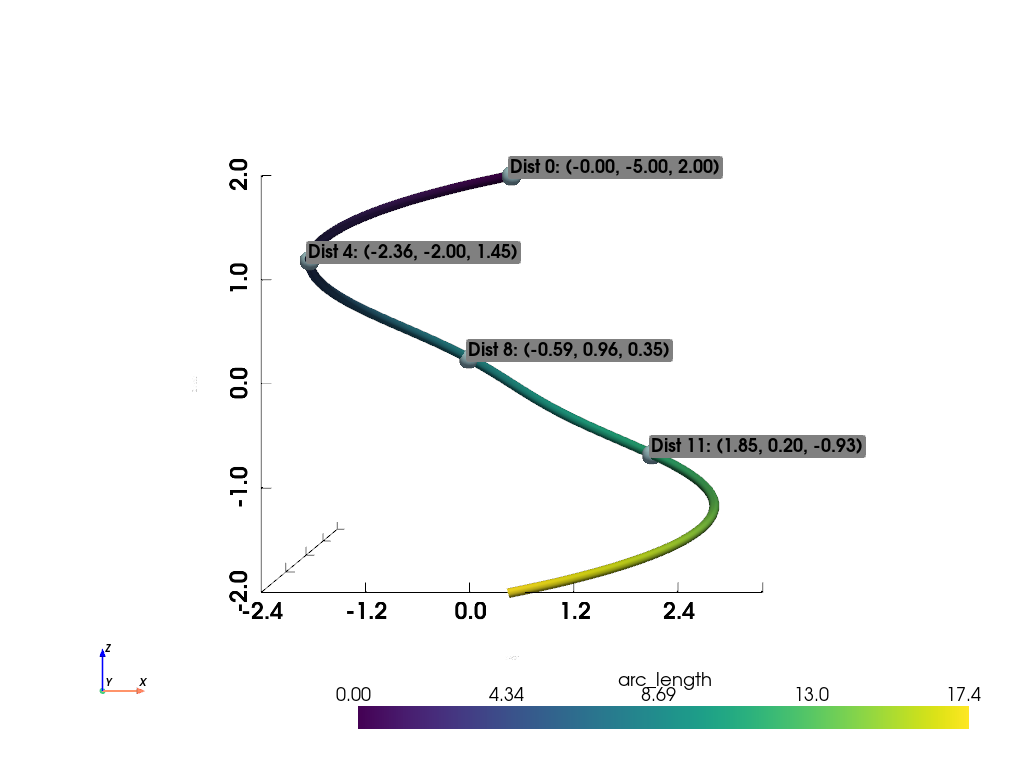
Total running time of the script: (0 minutes 0.431 seconds)