Note
Go to the end to download the full example code
Slicing#
Extract thin planar slices from a volume.
import matplotlib.pyplot as plt
import numpy as np
import pyvista as pv
from pyvista import examples
PyVista meshes have several slicing filters bound directly to all datasets. These filters allow you to slice through a volumetric dataset to extract and view sections through the volume of data.
One of the most common slicing filters used in PyVista is the
pyvista.DataSetFilters.slice_orthogonal()
filter which creates three
orthogonal slices through the dataset parallel to the three Cartesian planes.
For example, let’s slice through the sample geostatistical training image
volume. First, load up the volume and preview it:
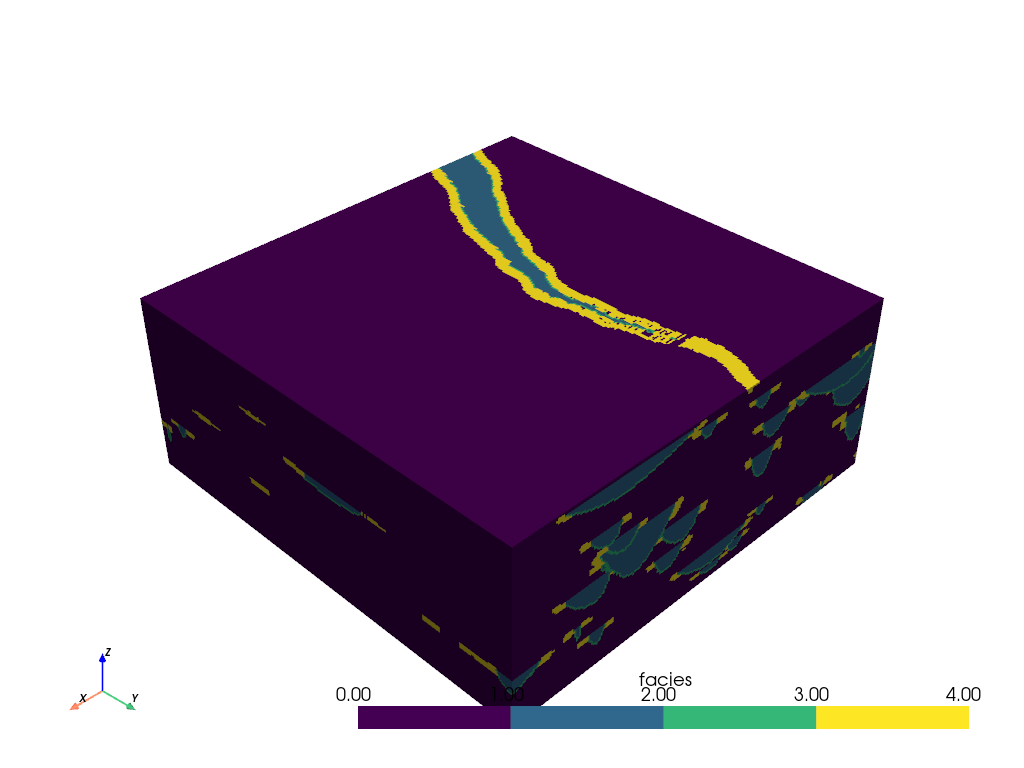
/home/runner/work/pyvista/pyvista/examples/01-filter/slicing.py:29: MatplotlibDeprecationWarning: The get_cmap function was deprecated in Matplotlib 3.7 and will be removed two minor releases later. Use ``matplotlib.colormaps[name]`` or ``matplotlib.colormaps.get_cmap(obj)`` instead.
cmap = plt.cm.get_cmap("viridis", 4)
Note that this dataset is a 3D volume and there might be regions within this volume that we would like to inspect. We can create slices through the mesh to gain further insight about the internals of the volume.
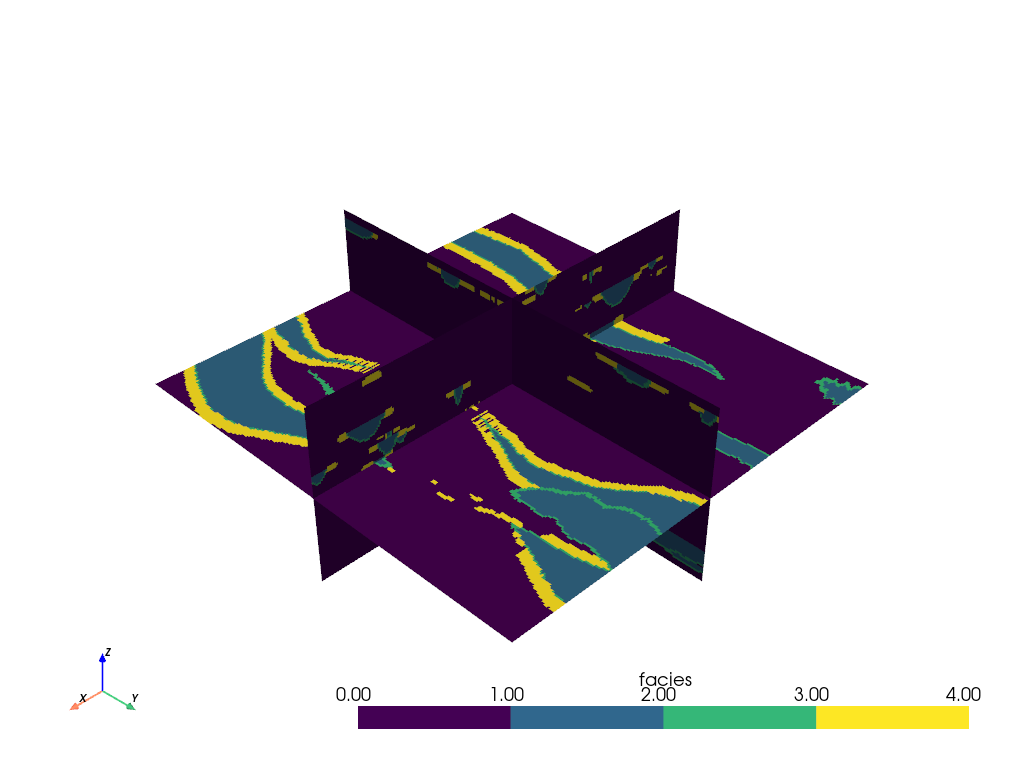
The orthogonal slices can be easily translated throughout the volume:
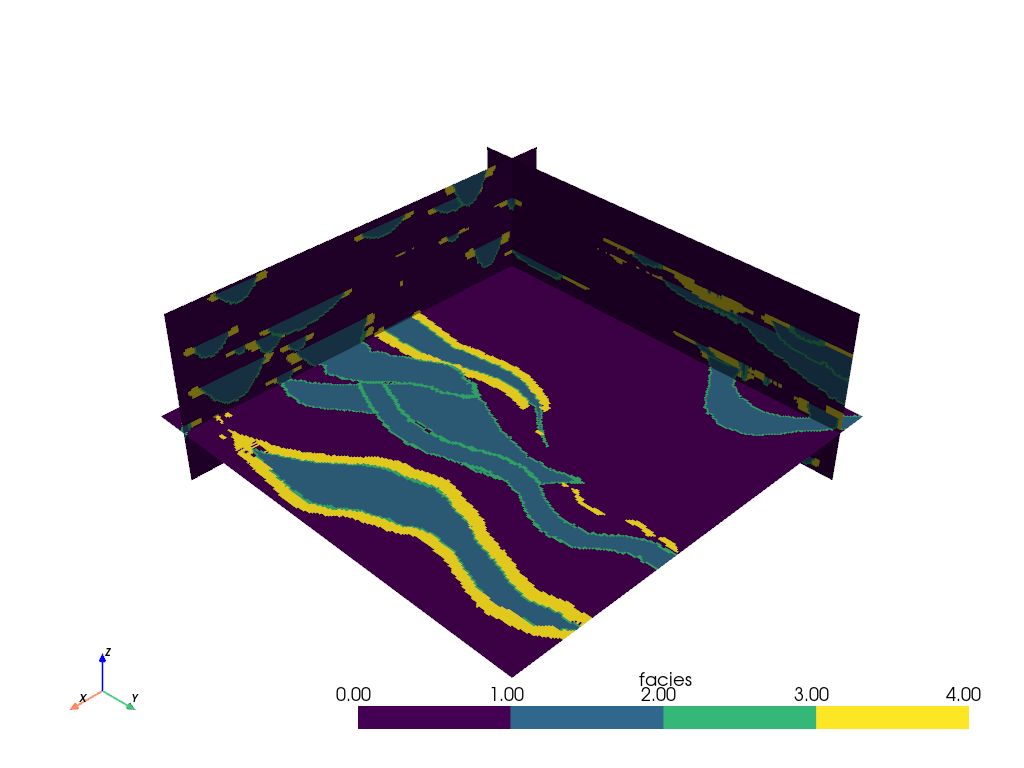
We can also add just a single slice of the volume by specifying the origin
and normal of the slicing plane with the pyvista.DataSetFilters.slice()
filter:
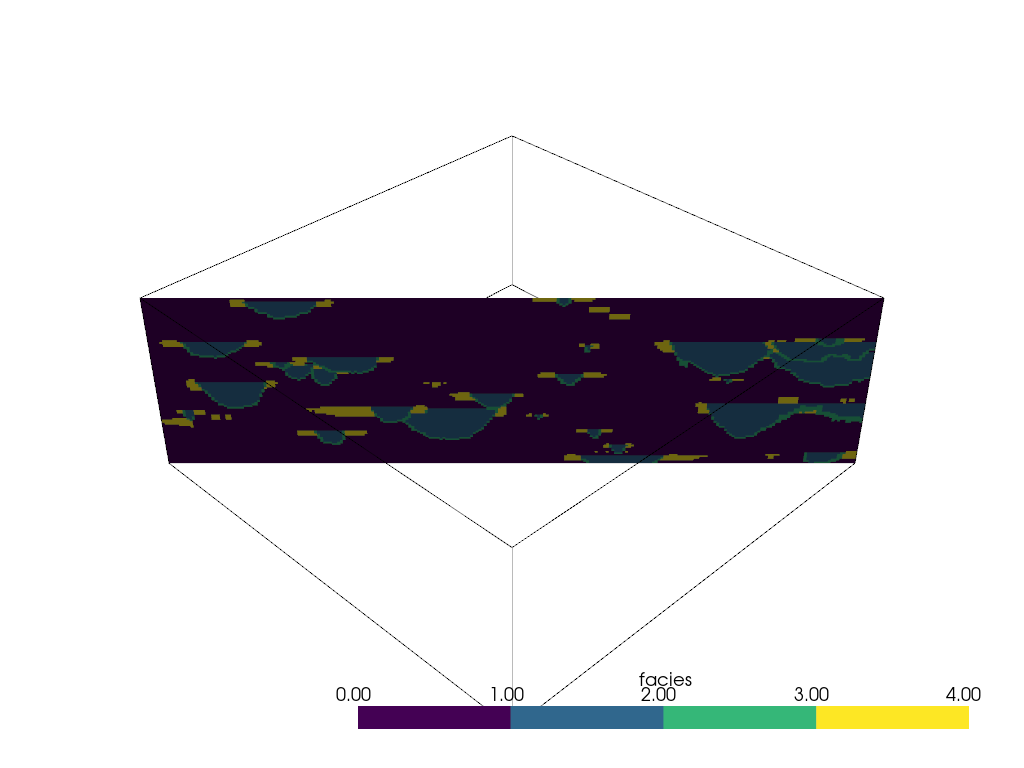
Adding slicing planes uniformly across an axial direction can also be
automated with the pyvista.DataSetFilters.slice_along_axis()
filter:
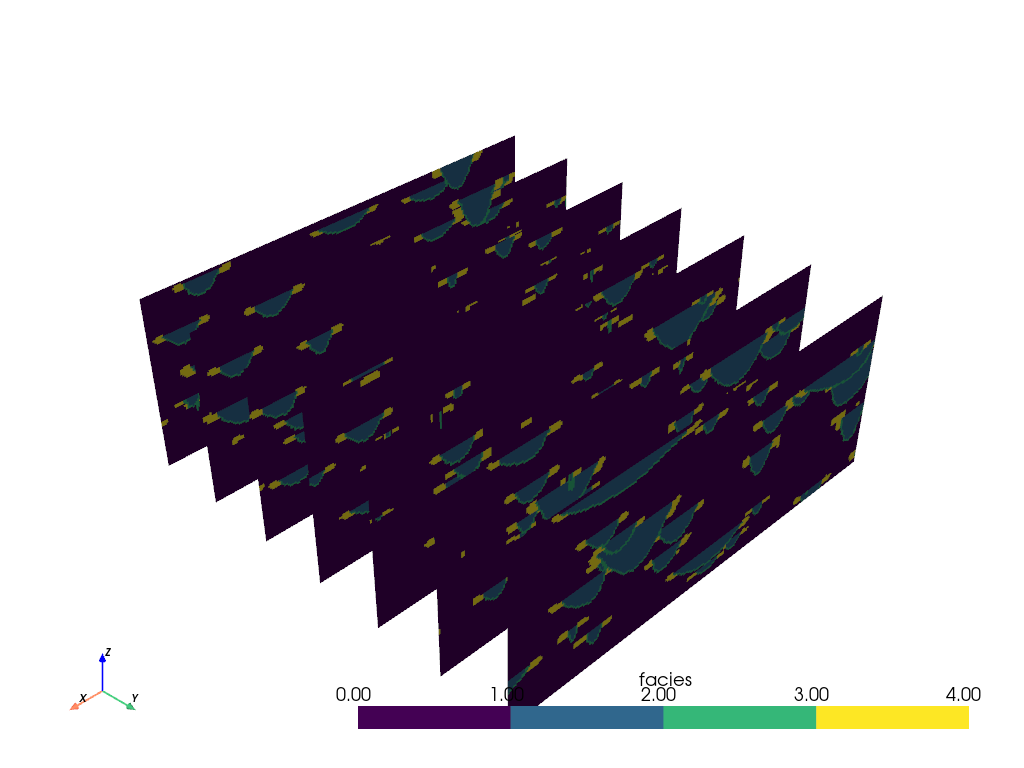
Slice Along Line#
We can also slice a dataset along a pyvista.Spline()
or
pyvista.Line()
using the pyvista.DataSetFilters.slice_along_line()
filter.
First, define a line source through the dataset of interest. Please note that this type of slicing is computationally expensive and might take a while if there are a lot of points in the line - try to keep the resolution of the line low.
Then run the filter
slc = model.slice_along_line(spline)
slc
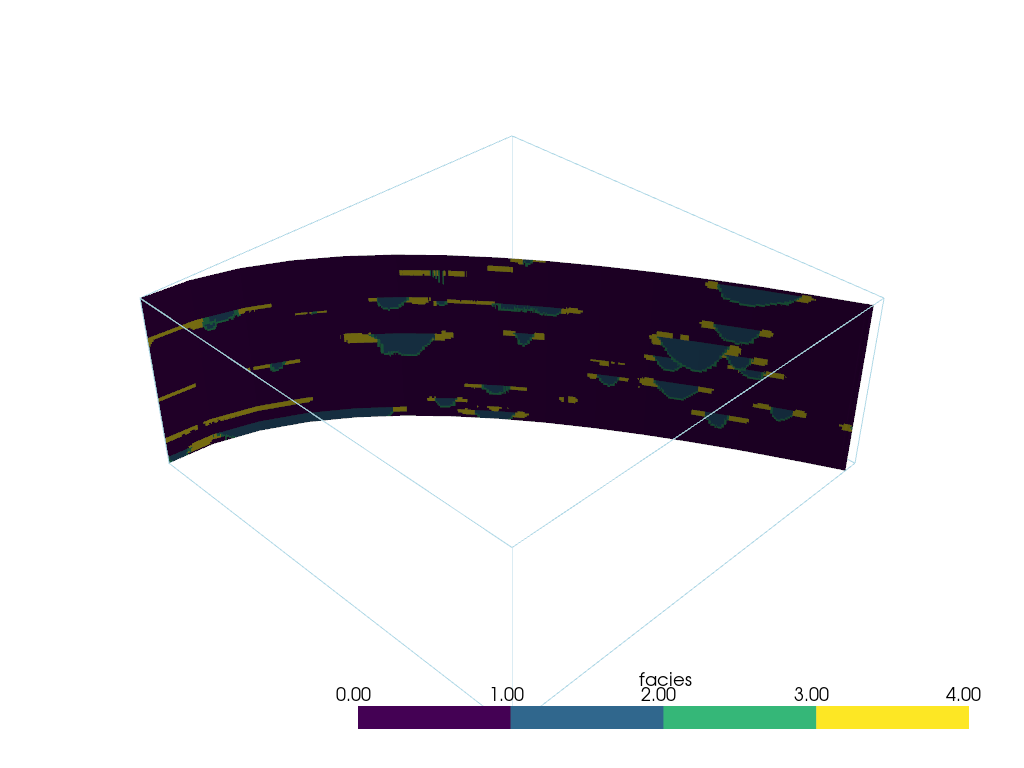
Multiple Slices in Vector Direction#
Slice a mesh along a vector direction perpendicularly.
mesh = examples.download_brain()
# Create vector
vec = np.array([1.0, 2.0, 1.0])
# Normalize the vector
normal = vec / np.linalg.norm(vec)
# Make points along that vector for the extent of your slices
a = mesh.center + normal * mesh.length / 3.0
b = mesh.center - normal * mesh.length / 3.0
# Define the line/points for the slices
n_slices = 5
line = pv.Line(a, b, n_slices)
# Generate all of the slices
slices = pv.MultiBlock()
for point in line.points:
slices.append(mesh.slice(normal=normal, origin=point))
p = pv.Plotter()
p.add_mesh(mesh.outline(), color="k")
p.add_mesh(slices, opacity=0.75)
p.add_mesh(line, color="red", line_width=5)
p.show()
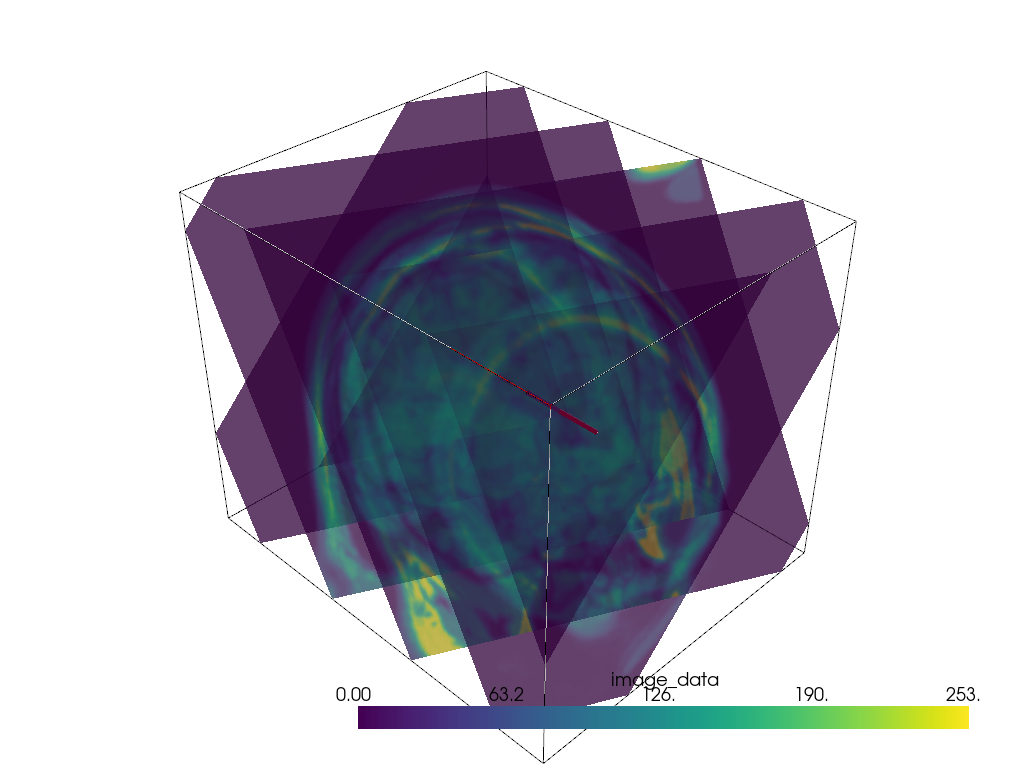
Slice At Different Bearings#
From pyvista-support#23
An example of how to get many slices at different bearings all centered around a user-chosen location.
Create a point to orient slices around
Now generate a few normal vectors to rotate a slice around the z-axis. Use equation for circle since its about the Z-axis.
increment = np.pi / 6.0
# use a container to hold all the slices
slices = pv.MultiBlock() # treat like a dictionary/list
for theta in np.arange(0, np.pi, increment):
normal = np.array([np.cos(theta), np.sin(theta), 0.0]).dot(np.pi / 2.0)
name = f'Bearing: {np.rad2deg(theta):.2f}'
slices[name] = model.slice(origin=point, normal=normal)
slices
And now display it.
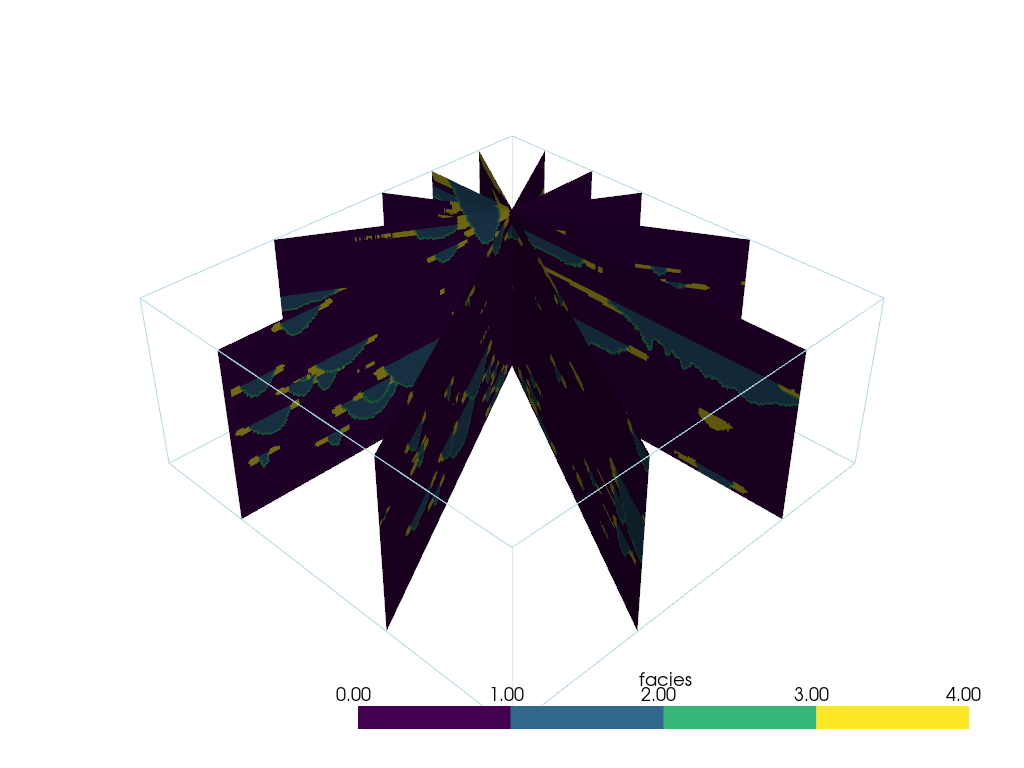
Total running time of the script: (0 minutes 16.431 seconds)