Composite Datasets#
The pyvista.MultiBlock
class is a composite class to hold many
data sets which can be iterated over. MultiBlock
behaves mostly like
a list, but has some Dictionary-like features.
List-like Features#
Create empty composite dataset
import pyvista as pv
from pyvista import examples
blocks = pv.MultiBlock()
blocks
Information | Blocks | |||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
|
Add some data to the collection.
blocks.append(pv.Sphere())
blocks.append(pv.Cube(center=(0, 0, -1)))
Plotting the MultiBlock
plots all the meshes contained by it.
blocks.plot(smooth_shading=True)
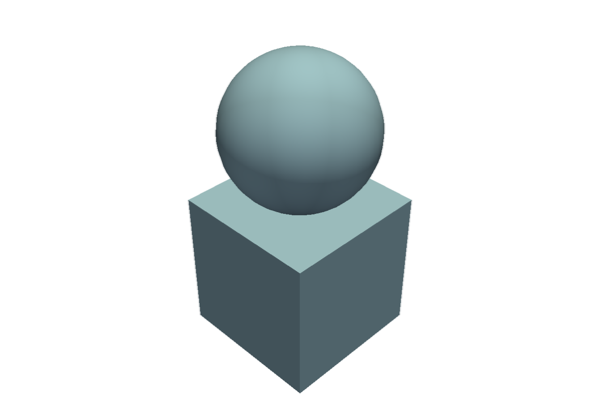
MultiBlock
is List-like, so individual blocks can be accessed via
indices.
blocks[0] # Sphere
Header | Data Arrays | ||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
|
The length of the block can be accessed through len()
len(blocks)
2
or through the n_blocks
attribute
blocks.n_blocks
2
More specifically, MultiBlock
is a collections.abc.MutableSequence
and supports operations such as append, pop, insert, etc. Some of these operations
allow optional names to be provided for the dictionary like usage.
blocks.append(pv.Cone(), name="cone")
cone = blocks.pop(-1) # Pops Cone
blocks.reverse()
MultiBlock
also supports slicing for getting or setting blocks.
blocks[0:2] # The Sphere and Cube objects in a new ``MultiBlock``
Information | Blocks | |||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
|
Dictionary-like Features#
MultiBlock
also has some dictionary features. We can set the name
of the blocks, and then access them
blocks = pv.MultiBlock([pv.Sphere(), pv.Cube()])
blocks.set_block_name(0, "sphere")
blocks.set_block_name(1, "cube")
blocks["sphere"] # Sphere
Header | Data Arrays | ||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
|
It is important to note that MultiBlock
is not a dictionary and does
not enforce unique keys. Keys can also be None
. Extra care must be
taken to avoid problems using the Dictionary-like features.
PyVista tries to keep the keys ordered correctly when doing list operations.
blocks.reverse()
blocks.keys()
['cube', 'sphere']
The dictionary like features are useful when reading in data from a file. The
keys are often more understandable to access the data than the index.
pyvista.examples.download_cavity()
is an OpenFoam dataset with a nested
MultiBlock
structure. There are two entries in the top-level object
data = examples.download_cavity()
data.keys()
['internalMesh', 'boundary']
"internalMesh"
is a pyvista.UnstructuredGrid
.
data["internalMesh"]
Header | Data Arrays | ||||||||||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
|
"boundary"
is another pyvista.MultiBlock
.
data["boundary"]
Information | Blocks | ||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
|
Using the dictionary like features of pyvista.MultiBlock
allow for easier
inspection and use of the data coming from an outside source. The names of each key
correspond to human understandable portions of the dataset.
data["boundary"].keys()
['movingWall', 'fixedWalls', 'frontAndBack']
Examples using this class:
MultiBlock API Reference#
The pyvista.MultiBlock
class holds attributes that
are common to all spatially referenced datasets in PyVista. This
base class is analogous to VTK’s vtk.vtkMultiBlockDataSet class.
|
A composite class to hold many data sets which can be iterated over. |